Introduction
LCD displays with Toshiba T6963C controller are ones of the most popular LCD displays. On this page You will find ready-to-use source code for Atmel AVR microcontrollers written in C language for avr-gcc compiler.
Connections between LCD and MCU are shown on below picture (it's only example, You can change ) :
Of course, this is very simple schematic without power lines and other required parts like crystal oscillator etc., which You must place in real design. But I think, that isn't problem for You.
I divide source code for following files :
- T6963C.h - configuration and functions declarations for T6963C.c file
- T6963C.c - all functions specific for T6963C controller
- graphic.c - functions for drawing lines, circles and rectangles (controller-independent)
- graphic.h - functions declarations for graphic.c file
All source codes are available in Download section. After download all files to folder with Your project and add T6963C.c and graphic.c files to project tree (or makefile) and place in file with the main() function calls to functions which You would to use.
For example, code shown below :
#include <avr/io.h>
#include "T6963C.h"
#include "graphic.h"
int main(void)
{
GLCD_Initalize(); // Initalize LCD
GLCD_ClearText(); // Clear text area
GLCD_ClearCG(); // Clear character generator area
GLCD_ClearGraphic(); // Clear graphic area
GLCD_TextGoTo(0,0);// set text coordinates
GLCD_WriteString("http://en.radzio.dxp.pl/t6963/"); // write text
GLCD_Circle(32,32,20); // draw circle
GLCD_Circle(208,32,20); // draw circle
GLCD_Rectangle(8,8,224, 48); // draw rectangle
while(1);
return 0;
} |
...will produced following image :
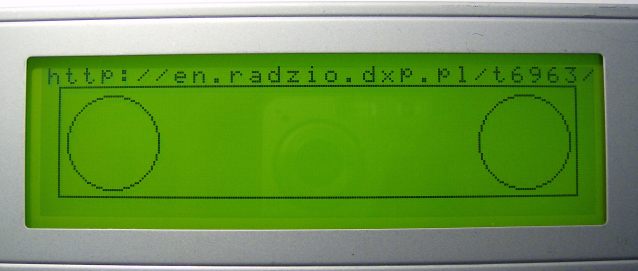
How to write inverted text?
Text inversion can be done by XORing text area with filled graphic area.
GLCD_WriteCommand(T6963_MODE_SET | 1); // enable XOR MODE
GLCD_TextGoTo(0, 0);
GLCD_WriteString("en.radzio.dxp.pl");
GLCD_FillRectangle(0, 0, 96, 8); // area that inverse text
GLCD_TextGoTo(0, 1);
GLCD_WriteString("en.radzio.dxp.pl"); |
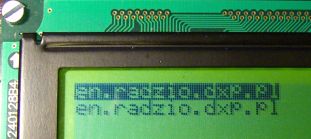
For drawing filled rectangle use this function :
void GLCD_FillRectangle(uint8_t x, uint8_t y, uint8_t dx, uint8_t dy)
{
uint8_t i, j;
for(i = x; i < x + dx; i++)
for(j = y; j < y + dy; j++)
GLCD_SetPixel(i, j, 1);
}
|
Download
Source code for avr-gcc compiler. It can be ported for others compilers and MCU's.
Source code for AT91SAM ARM based devices is on this page : AT91 T6963C
See also : Universal library for SED1335 , ATmega162 + external RAM
|